Implementation of input stream based on a memory chunk. More...
#include <SFML/System/MemoryInputStream.hpp>
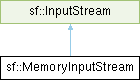
Public Member Functions | |
MemoryInputStream (const void *data, std::size_t sizeInBytes) | |
Construct the stream from its data. | |
std::optional< std::size_t > | read (void *data, std::size_t size) override |
Read data from the stream. | |
std::optional< std::size_t > | seek (std::size_t position) override |
Change the current reading position. | |
std::optional< std::size_t > | tell () override |
Get the current reading position in the stream. | |
std::optional< std::size_t > | getSize () override |
Return the size of the stream. | |
Detailed Description
Implementation of input stream based on a memory chunk.
This class is a specialization of InputStream
that reads from data in memory.
It wraps a memory chunk in the common InputStream
interface and therefore allows to use generic classes or functions that accept such a stream, with content already loaded in memory.
In addition to the virtual functions inherited from InputStream
, MemoryInputStream
adds a function to specify the pointer and size of the data in memory.
SFML resource classes can usually be loaded directly from memory, so this class shouldn't be useful to you unless you create your own algorithms that operate on an InputStream.
Usage example:
- See also
InputStream
,FileInputStream
Definition at line 46 of file MemoryInputStream.hpp.
Constructor & Destructor Documentation
◆ MemoryInputStream()
sf::MemoryInputStream::MemoryInputStream | ( | const void * | data, |
std::size_t | sizeInBytes ) |
Construct the stream from its data.
- Parameters
-
data Pointer to the data in memory sizeInBytes Size of the data, in bytes
Member Function Documentation
◆ getSize()
|
overridevirtual |
Return the size of the stream.
- Returns
- The total number of bytes available in the stream, or
std::nullopt
on error
Implements sf::InputStream.
◆ read()
|
nodiscardoverridevirtual |
Read data from the stream.
After reading, the stream's reading position must be advanced by the amount of bytes read.
- Parameters
-
data Buffer where to copy the read data size Desired number of bytes to read
- Returns
- The number of bytes actually read, or
std::nullopt
on error
Implements sf::InputStream.
◆ seek()
|
nodiscardoverridevirtual |
Change the current reading position.
- Parameters
-
position The position to seek to, from the beginning
- Returns
- The position actually sought to, or
std::nullopt
on error
Implements sf::InputStream.
◆ tell()
|
nodiscardoverridevirtual |
Get the current reading position in the stream.
- Returns
- The current position, or
std::nullopt
on error.
Implements sf::InputStream.
The documentation for this class was generated from the following file: