Implementation of input stream based on a file. More...
#include <SFML/System/FileInputStream.hpp>
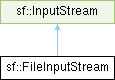
Public Member Functions | |
FileInputStream () | |
Default constructor. | |
~FileInputStream () override | |
Default destructor. | |
FileInputStream (const FileInputStream &)=delete | |
Deleted copy constructor. | |
FileInputStream & | operator= (const FileInputStream &)=delete |
Deleted copy assignment. | |
FileInputStream (FileInputStream &&) noexcept | |
Move constructor. | |
FileInputStream & | operator= (FileInputStream &&) noexcept |
Move assignment. | |
FileInputStream (const std::filesystem::path &filename) | |
Construct the stream from a file path. | |
bool | open (const std::filesystem::path &filename) |
Open the stream from a file path. | |
std::optional< std::size_t > | read (void *data, std::size_t size) override |
Read data from the stream. | |
std::optional< std::size_t > | seek (std::size_t position) override |
Change the current reading position. | |
std::optional< std::size_t > | tell () override |
Get the current reading position in the stream. | |
std::optional< std::size_t > | getSize () override |
Return the size of the stream. | |
Detailed Description
Implementation of input stream based on a file.
This class is a specialization of InputStream
that reads from a file on disk.
It wraps a file in the common InputStream
interface and therefore allows to use generic classes or functions that accept such a stream, with a file on disk as the data source.
In addition to the virtual functions inherited from InputStream
, FileInputStream
adds a function to specify the file to open.
SFML resource classes can usually be loaded directly from a filename, so this class shouldn't be useful to you unless you create your own algorithms that operate on an InputStream.
Usage example:
- See also
InputStream
,MemoryInputStream
Definition at line 56 of file FileInputStream.hpp.
Constructor & Destructor Documentation
◆ FileInputStream() [1/4]
sf::FileInputStream::FileInputStream | ( | ) |
Default constructor.
Construct a file input stream that is not associated with a file to read.
◆ ~FileInputStream()
|
override |
Default destructor.
◆ FileInputStream() [2/4]
|
delete |
Deleted copy constructor.
◆ FileInputStream() [3/4]
|
noexcept |
Move constructor.
◆ FileInputStream() [4/4]
|
explicit |
Construct the stream from a file path.
- Parameters
-
filename Name of the file to open
- Exceptions
-
sf::Exception on error
Member Function Documentation
◆ getSize()
|
overridevirtual |
Return the size of the stream.
- Returns
- The total number of bytes available in the stream, or
std::nullopt
on error
Implements sf::InputStream.
◆ open()
|
nodiscard |
Open the stream from a file path.
- Parameters
-
filename Name of the file to open
- Returns
true
on success,false
on error
◆ operator=() [1/2]
|
delete |
Deleted copy assignment.
◆ operator=() [2/2]
|
noexcept |
Move assignment.
◆ read()
|
nodiscardoverridevirtual |
Read data from the stream.
After reading, the stream's reading position must be advanced by the amount of bytes read.
- Parameters
-
data Buffer where to copy the read data size Desired number of bytes to read
- Returns
- The number of bytes actually read, or
std::nullopt
on error
Implements sf::InputStream.
◆ seek()
|
nodiscardoverridevirtual |
Change the current reading position.
- Parameters
-
position The position to seek to, from the beginning
- Returns
- The position actually sought to, or
std::nullopt
on error
Implements sf::InputStream.
◆ tell()
|
nodiscardoverridevirtual |
Get the current reading position in the stream.
- Returns
- The current position, or
std::nullopt
on error.
Implements sf::InputStream.
The documentation for this class was generated from the following file: